These architecture patterns are among the most commonly used in app development, whether on iOS or Android platforms. Developers have introduced them to overcome the limitations of earlier patterns. So, how do they differ?
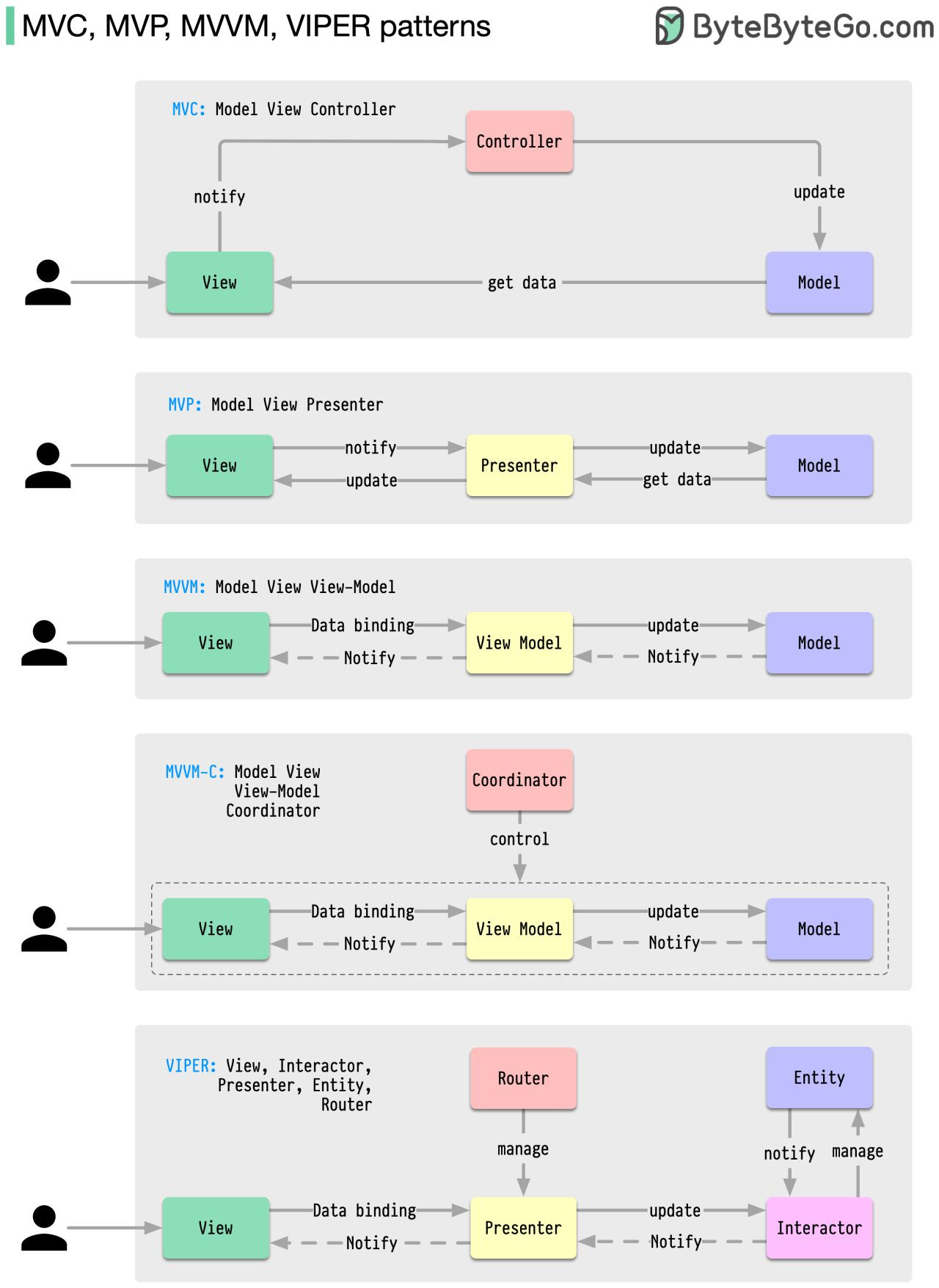
- MVC, the oldest pattern, dates back almost 50 years
- Every pattern has a “view” (V) responsible for displaying content and receiving user input
- Most patterns include a “model” (M) to manage business data
- “Controller,” “presenter,” and “view-model” are translators that mediate between the view and the model (“entity” in the VIPER pattern)
- These translators can be quite complex to write, so various patterns have been proposed to make them more maintainable
MVC, MVP, MVI, MVVM and VIPER Design Patterns
“In the last couple of years, several new patterns emerged, all praising to make a developer’s life as easy as never before. By separating certain parts of the codebase, each of these patterns tries to make code more readable, easier to test, and eventually more maintainable.”
In this article I am going to talk about software architecture design patterns, MVC, MVP, MVI, MVVM and VIPER.
What is software architecture?
A software project needs to be planned before it is development. This planning is called “Software Architecture” and the people who designed this plan are called “Software Architects”.
In other words, software architecture is the process of converting software characteristics into a structured solution that fulfills your business needs and technical requirements.
Thus, the path to be followed by software developers is determined in general terms.
What about design patterns?
In general, it helps to modularize the software so that each component is separate and fulfills a single responsibility. It means, design patterns have always helped to create manageable, testable, reusable and optimized software.
They also increase the readability of the code and software development process is greatly accelerated with them.
MVC (Model-View-Controller)
MVC is the most well-known software architecture. It is based on the abstraction of data and data representation in complex applications where a large amount of data is presented to the user. Thus, data (model) and user interface (view) can be edited without affecting each other.
Thanks to MVC, we can develop and test our code more easily.
The biggest advantage of MVC is that it cleanly distributes responsibilities to Model, View and Controller.
It contains 3 sections; model, view, controller. Let’s examine these 3 sections in detail.
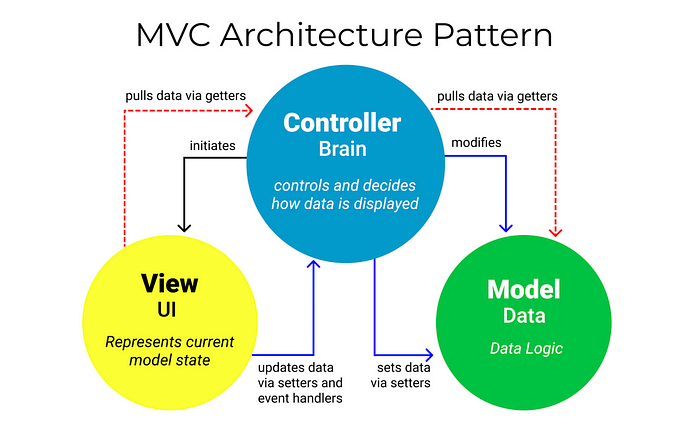
Model:
“The backend that contains all the data logic”
- Where the data is stored.
- Model isolates the data layer from the application.
View:
“The frontend or graphical user interface (GUI)”
- View only contains the business of how to update itself, creates the Model and displays it to the user.
- There can be multiple Views using the same controller for different platforms as the View does not contain any application-related logic.
- The Controller must update the Model for the View to be updated.
Controller:
“The brains of the application that controls how data is displayed”
- Meets the inputs from the user and makes changes on the Model in line with the inputs from the user.
- It also manages the whole flow and makes the decisions regarding the UI.
- Controller knows nothing about View but View knows Controller.
- A Controller can be used by more than one View.
In addition, when MVC is applied, we may have difficulties in Unit Testing, the process related to the update of the View is done quite indirectly, there is no relationship between the Controller and the View. Even with an abstraction, we cannot Mock the Views.
MVP (Model-View-Presenter)

MVP Pattern is actually an evolved pattern from MVC, only dependencies are changing and Controller is replaced by Presenter.
- Model is same as in MVC Model
- View meets the inputs from the user
- View knows Presenter, Presenter knows View through an interface, there is an abstraction between them.
- Unlike MVC, there is a 1–1 relationship between View and Presenter.
- The Presenter doesn’t care what the View is, it just knows what to do with the data flow, how to handle the interactions from the View, and how to make changes to the View.
- In MVP, views can be mocked very easily, which makes Unit testing easier unlike in MVC.
- The UI independence principle can be applied more easily in applications written with MVP.
The biggest difference between MVP and MVC is that Presenter updates the View via an interface, where View can open the information it wants to Presenter through the interface.
Martin Fowler handles MVP with 2 different approaches; Passive View and Supervising Controller. I recommend that you read about them. 🙂
MVVM (Model-View-ViewModel)
Before giving information about the MVVM Pattern, I would like to give information about the Presentation Pattern.
Presentation model:
- The schema is a bit like MVP but the difference here is that the Presentation Model both keeps the state related to the View and doesn’t know anything about the View.
- View still keeps states. But unlike MVP, it doesn’t need any information about Presentation Model View.
- The disadvantage is that on platforms that do not have any Binding infrastructure, it may be necessary to write a lot of code to ensure synchronization between PM and View.

The biggest benefit of MVVM to software developers is Separation of Concerns. In other words, by separating the structures in our application from each other, it enables us to construct a less connected structure, and separates components such as visual interface and business structures in the background, data access structures. In this way, software developers and interface designers have the opportunity to work independently on the same project simultaneously.
Model represents our data coming from database, web services or any data source. In addition, business rules that control data consistency and accuracy are also included here.
View is the visual interface where we transfer our data to end users. It acts as a bridge between the end user and the application.
On the other hand, ViewModel acts as a bridge between visual interface and model. It is the structure that connects the Model to the View. There is no direct interaction between View and Model. View performs related operations via ViewModel. The ViewModel does not have direct access to the View and does not know anything about the View.
MVI (Model-View-Intent)
“MVP and MVVM are already great patterns for projects of any size, with MVC rarely being used in projects any bigger than small apps, but none of them was built with the reactive concept in mind.”
“MVI was designed with reactive programming in mind.”

The Model represents a situation. Models in MVI must be immutable to allow a one-way flow of data between them and between other layers of the architecture.
As in MVP, interfaces in MVI represent Views that are then implemented in one or more activities or fragments.
Intent represents the intention or willingness to perform an action by the user or the application itself. For every action, a view takes an intent. The Presenter observes the Intent and Models it to a new state.
“The first thing that stands out is the lack of our model. The reason for that is that the MVI models are states on their own. Instead of having a ViewModel notify our view about new models, our model itself is the state.”
VIPER (View-Interactor-Presenter-Entity-Router)
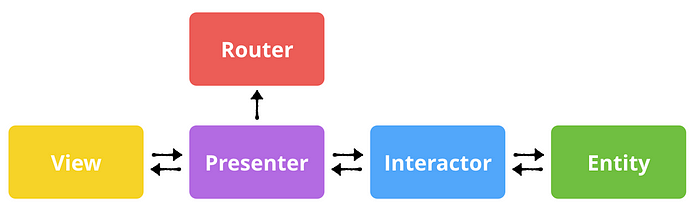
View: Here we only use the data from the presenter. No other action is taken.
Interactor: We can say that it acts as the VM in the MVVM design pattern. It is the part of the application that we call Bussines Logic. UI operations are not performed here. Fetch, Update etc. operations take place here.
Presenter: It is the bridge between View and Interactor. This layer should not contain UI or bussines logic operations.
Entity: It is the Model part of the application. Data models related to the application are found here. This part cooperates only with Interactor.
Router: This layer is the layer that allows us to determine when the application’s pages are shown.
Note that this architecture also has its own disadvantages such as difficulty in using it in small projects and causing complexity because of many folders and delegates in the project.